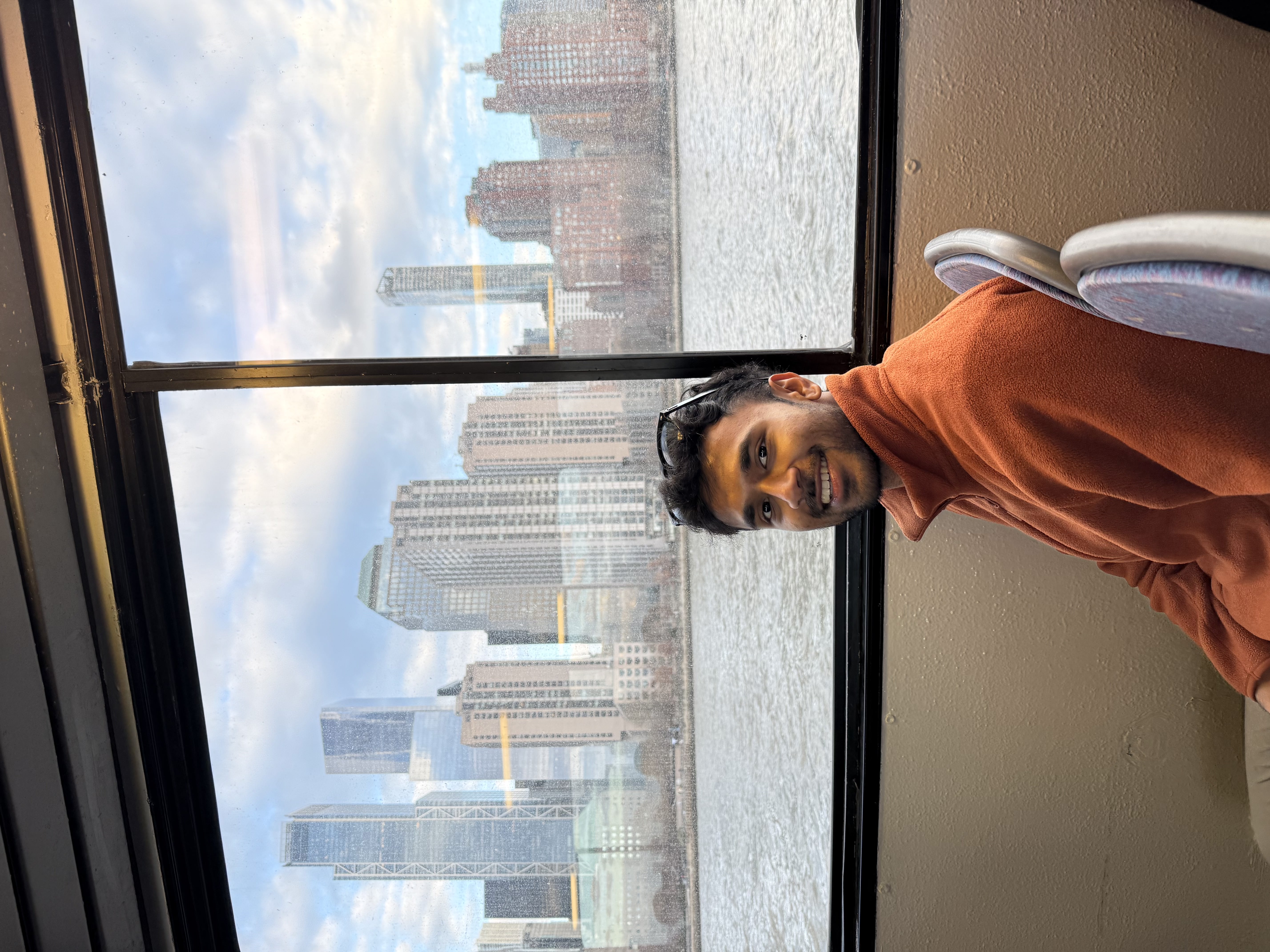
Apache Spark has become the go-to framework for building efficient, distributed data processing pipelines. However, when it comes to real-time data processing, there are several optimizations that can significantly improve performance. In this article, we'll explore how to fine-tune your Spark ETL pipelines for maximum efficiency.
Understanding the Challenges of Real-time ETL
Real-time ETL presents unique challenges compared to batch processing. The data arrives continuously, and the processing system needs to maintain low latency while ensuring data consistency and fault tolerance. These requirements put additional strain on the processing framework.
Here are some common challenges with real-time ETL:
- Maintaining low latency as data volume grows
- Handling late-arriving data
- Managing stateful operations across distributed processes
- Dealing with backpressure when downstream systems can't keep up
- Ensuring exactly-once processing semantics
Key Optimizations for Spark Streaming ETL
1. Tune Micro-batch Duration
Spark Structured Streaming processes data in micro-batches. The batch duration is a critical parameter that impacts both latency and throughput:
// Setting trigger interval to 1 second
spark
.readStream
.format("kafka")
.option("kafka.bootstrap.servers", "localhost:9092")
.option("subscribe", "input-topic")
.load()
.writeStream
.trigger(Trigger.ProcessingTime("1 second"))
.format("console")
.start()
Shorter intervals reduce latency but increase overhead. Longer intervals improve throughput but increase end-to-end latency. I recommend starting with a 1-second interval and adjusting based on your specific use case.
2. Optimize Partition Count
The number of partitions affects parallelism in Spark. Too few partitions limit parallelism, while too many increase overhead. As a rule of thumb, aim for 2-3 partitions per CPU core:
// Repartitioning data to optimize parallelism
streamingDF
.repartition(numPartitions)
.writeStream
.format("parquet")
.start()
3. Implement Windowed Operations Efficiently
Windowed operations are common in real-time processing but can be resource-intensive. Optimize them by:
- Using sliding windows only when necessary
- Setting appropriate watermarks to handle late data and limit state size
- Minimizing the window duration to reduce state size
// Setting a watermark and defining a window
streamingDF
.withWatermark("eventTime", "10 minutes")
.groupBy(window($"eventTime", "5 minutes"))
.agg(count("*").as("events"))
4. Leverage Checkpointing
Checkpointing is crucial for fault tolerance in streaming applications, but it can also impact performance:
// Configure checkpointing
streamingQuery
.option("checkpointLocation", "/path/to/checkpoint")
.start()
Store checkpoints on a reliable, high-speed storage system. For production, I recommend using an HDFS-compatible distributed file system or cloud storage like S3.
Real-world Patterns for Scalable ETL
Pattern 1: The Lambda Architecture
Combining batch and streaming processing can provide both accuracy and speed. In this pattern:
- The batch layer processes historical data for completeness
- The speed layer handles real-time data for low latency
- The serving layer combines results from both layers
Pattern 2: Stateless Transformations
Whenever possible, design transformations to be stateless. Stateless operations can be parallelized more efficiently and reduce memory pressure. Push stateful operations downstream when possible.
Pattern 3: Data Validation at Ingestion
Filtering and validating data early in the pipeline prevents invalid records from consuming resources:
// Filter invalid records early
streamingDF
.filter($"value".isNotNull && length($"value") > 0)
.filter(/* business validation logic */)
.select(/* transformations */)
Conclusion
Optimizing Spark ETL pipelines for real-time processing requires a combination of understanding Spark's execution model, tuning configuration parameters, and implementing efficient design patterns. By applying the techniques described in this article, you can significantly improve the performance, reliability, and scalability of your real-time data processing workflows.
In my next post, I'll dive deeper into monitoring and troubleshooting Spark Streaming jobs in production environments. Stay tuned!