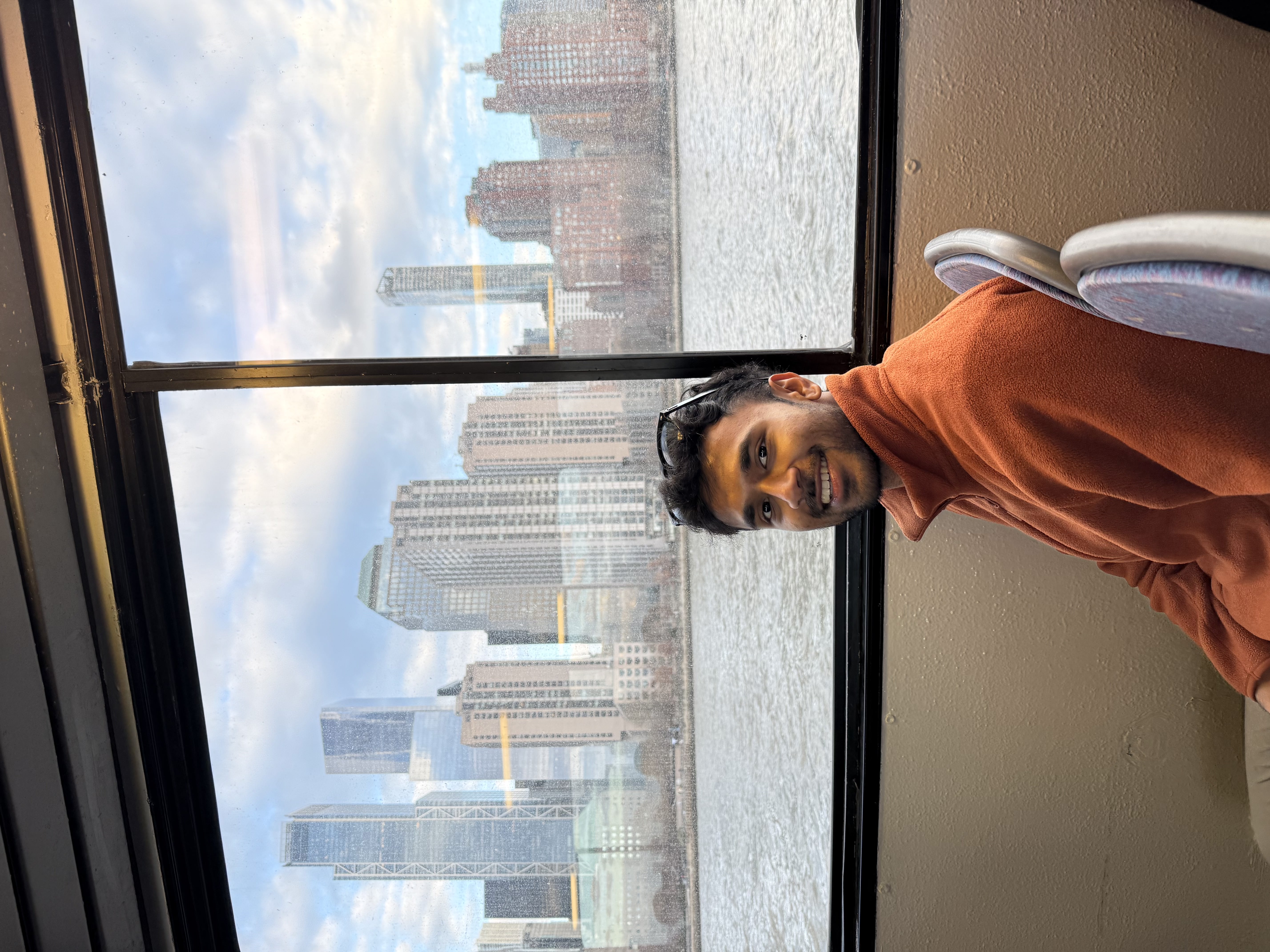
Imagine merging a pull request and—like magic—your new AWS Lambda function is tested, packaged, and deployed to production without you lifting a finger. Welcome to fully serverless CI/CD! In this post, we'll build an end-to-end pipeline using AWS CodeCommit, CodeBuild, CodeDeploy, and orchestrate it all with Step Functions for transparency, retries, and easy observability.
Why Go Serverless for CI/CD?
Traditional CI/CD servers are great, but they come with management overhead and scaling concerns. By using AWS’s managed services, you pay only when your pipeline runs, eliminate server maintenance, and leverage built-in integrations with IAM, CloudWatch, and EventBridge for notifications and monitoring.
Architecture Overview
Our pipeline will consist of:
- CodeCommit: Git repo for your Lambda code.
- CodeBuild: Runs unit tests and packages your function into a .zip.
- CodeDeploy: Deploys the package to your Lambda alias with traffic shifting.
- Step Functions: Coordinates each step, handles errors, and sends notifications.
This modular design means you can swap in third-party tools or add approval steps with minimal changes.
Step-by-Step Guide
1. Create Your CodeCommit Repository
In the AWS Console, navigate to CodeCommit and create a new repo called lambda-app
. Clone it locally and add your Lambda handler, a buildspec.yml
, and a unit test folder:
git clone https://git-codecommit.us-east-1.amazonaws.com/v1/repos/lambda-app
cd lambda-app
# Add your lambda_function.py, tests/, buildspec.yml
git add .
git commit -m "Initial commit"
git push origin master
2. Define Your Build Specification
Your buildspec.yml
should install dependencies, run tests, and create an artifact:
version: 0.2
phases:
install:
commands:
- pip install -r requirements.txt -t .
build:
commands:
- pytest tests/
artifacts:
files:
- '**/*'
discard-paths: yes
base-directory: .
3. Setup CodeBuild Project
Create a CodeBuild project linked to your repo. Choose the managed image aws/codebuild/standard:5.0
, enable buildspec from source, and give it a service role with S3 read/write (for artifacts) and permissions to invoke Step Functions.
4. Configure CodeDeploy for Lambda
Use AppSpec
for Lambda in your repo. In appspec.yml
:
version: 0.0
Resources:
- myLambdaFunction:
Type: AWS::Lambda::Function
Properties:
Name: my-lambda-app
Alias: live
Hooks:
- BeforeAllowTraffic: validate
- AfterAllowTraffic: notify
Create a CodeDeploy application of type “Lambda” and a deployment group targeting your function’s alias with a linear traffic shift of 10% every minute.
5. Orchestrate with Step Functions
In Step Functions, define a state machine that:
- Starts the CodeBuild job.
- Waits for build success or failure.
- On success, triggers a CodeDeploy deployment.
- Monitors deployment status.
- Sends success/failure notifications via SNS or ChatOps.
Your stateMachine.asl.json
might look like:
{
"StartAt": "Build",
"States": {
"Build": {
"Type": "Task",
"Resource": "arn:aws:states:::codebuild:startBuild.sync",
"Parameters": { "ProjectName": "lambda-app-build" },
"Next": "Deploy"
},
"Deploy": {
"Type": "Task",
"Resource": "arn:aws:states:::codedeploy:createDeployment",
"Parameters": {
"ApplicationName": "lambda-app-cd",
"DeploymentGroupName": "LambdaAliasGroup",
"Revision": { /* S3 location from build */ }
},
"Next": "WaitForDeployment"
},
"WaitForDeployment": {
"Type": "Task",
"Resource": "arn:aws:states:::codedeploy:getDeployment",
"Parameters": { "deploymentId.$": "$.deploymentId" },
"End": true
}
}
}
Testing & Monitoring
Once deployed, commit a small change, push to CodeCommit, and watch your pipeline in the Step Functions console. You’ll see each step light up, know exactly where failures happen, and can retry individual states. CloudWatch logs, metrics, and X-Ray traces (if enabled) give deep insights into performance.
Conclusion
With this serverless CI/CD approach, you get zero-ops infrastructure, pay-per-execution, and a transparent, reliable pipeline. Whether you’re a one-person team or a large organization, automating Lambda releases with Step Functions scales with you. Happy deploying!
Next up: securing your pipeline with least-privilege roles and integrating approval gates via AWS Lambda and API Gateway. Stay tuned!